Even though Microsoft has explicitly told the world to stop using Internet Explorer 11, many organizations still have a significant enough IE11 user base that they have no choice but to build their products to acquiesce to the aging browser’s idiosyncratic demands.
Whether you are new to the world of building the front-end for Internet Explorer 11 compatibility or you are an old hand who knows their way around all the IE11 pitfalls, read on to learn more about:
- Choosing the right environment for debugging
- Dealing with unsupported JavaScript
- Tackling CSS layout issues
- Understanding the IE11 debugger
Armed with these tips, one should be able to quickly solve problems in the instance when a web application works perfectly on everything but IE11.
Choosing an Environment
The fastest and most efficient way to debug on IE11 is to open up the browser on a Windows machine. This is my personal preference at the moment (though it can always change), and since I have a spare computer running Windows, I’m able to do this. For those of us who don’t have access to a Windows machine, there are a few options available.
BrowserStack
Here at Chromatic, we are a (mostly) macOS shop, so we use BrowserStack for testing on multiple environments and browser versions. If you haven’t used it before, BrowserStack allows you to preview your sites and web applications in various browsers and platforms, including real mobile devices all through your browser window. We rely on it a lot, but it can lag at certain times. This adds to the amount of time it takes to solve a problem, which ultimately does not help in delighting our clients.
Virtual Environment
When I find that BrowserStack is running slow, I end up using a virtual environment. There are plenty of options out there, but I’ve found VirtualBox to be the easiest to set up. The most straightforward set of instructions on how to set up Windows 10 that I found was on an article over at Viget and I recently tested it out myself to great success. With this setup, I’ve been able to access my local environment (by typing out http://10.0.2.2:3000
instead of localhost:3000
) without taking any additional steps. However, if you have any trouble, you can refer to these great troubleshooting tips on Medium. While on the Windows VM, I was also able to route through a VPN tunnel when the VPN was active from the Host by reconfiguring the VirtualBox NAT Networking settings.
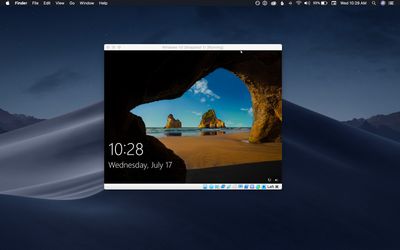
VirtualBox provides a straightforward way to run Windows 10 on Mac
Note: If you are interested in more detailed, step-by-step instructions on VPN access through a VirtualBox VM, tweet us @chromatichq and we can whip up a quick post on the matter.
Other than VirtualBox, another option to consider is Vagrant, which is a powerful tool “for building and maintaining portable virtual software development environments.” One of my colleagues uses Vagrant when running Windows in a virtual environment and recommends it. I found that going down this route does require several extra steps and could potentially take more time than a VirtualBox setup, but after you successfully vagrant up
, you can reap the many benefits of Vagrant’s power and flexibility.
Breaking down the error
The two most common issues I’ve run into when finding that an application in IE11 does not look or behave properly are:
<insert modern ES6 syntax here> is undefined
issues- Layout style issues
Support for ES6, ES7 and Beyond
If QA has flagged unexpected behavior after some type of user interaction, but you do not see any issues in Chrome or Firefox, there is a good chance it may be a modern JavaScript compatibility issue. In my experience, this has been the #1 most common issue I’ve seen. If you are expected to develop for IE11 compatibility (read on regarding setting developer expectations for IE11), then be aware that while Microsoft has promised that the browser will continue to receive security updates, it has no plans to continue adding any new features to IE11.
However, this doesn’t mean that one should avoid using ES6 and beyond. It’s important to continue using improved-upon programming languages that are easier to write and more powerful. Since it will eventually run in all browsers, it’s important to keep up with modern practices. If you are working on any type of modern web application, you are likely already running a transpiler like Babel, which converts your ECMAScript 2015+ code into a backwards-compatible version of JavaScript in older browsers.
But what if you have Babel running, and you’re still getting errors in IE11 such as Array.from is undefined
? Babel won't transpile Array.from
, so you will have to load a polyfill to handle it.
There are two ways to approach this. First, you can update your configuration. Babel 7.4 uses the newest version of core-js@3
to handle polyfills and there are step-by-step instructions on how to update core-js
and set your versions.
Second, if you are not keen on installing any more packages and adding unnecessary scripts that you may never need, we’ve found that manually adding a specific polyfill somewhere in your JavaScript or creating a custom JS file specifically for polyfills is also appropriate.
One popular tool is Juriy Zaytsev’s compat-table, which organizes JavaScript syntax, bindings, functions, and built-ins to see what works where.
In this example, you can quickly check the limitations of Symbol if you decide you want to use it in your code and you are on a specific version of Babel:
Common CSS Issues in IE11
When styling elements, I often use CSS Flexbox for laying out items in rows and columns, and I’ve found that it’s useful for forcing items to either fill additional space, or shrink to fit into smaller spaces. It is popular because it is lightweight and intuitive, but it can also cause unpredictable behavior when dealing with IE11. If you decide to use flex
on an element, it may be a good idea to keep IE11 open when adding in the flexbox-related style rules so there are no surprises and you can track changes.
One problem I ran into recently in IE11 was that min-height:
worked on the parent element, but the flex-item child behaved as if no height had been set. The workaround for me was to use another wrapper around the parent container that is also a flex container.
There’s a great GitHub project called Flexbugs that details all the known issues.
How to Debug in Internet Explorer 11 (IE11)
Sometimes, you have no choice but to use the IE11 debugger, especially when you see an error on the console that you do not recognize. Since there seems to be limited documentation on the matter, the following are a list of detailed steps I recommend when stepping through errors in IE11.
Step 1
To save you some sanity, make sure you disable auto-debugging, which forces you to break on all exceptions in Internet Explorer 11. That way, after you adjust the settings in your browser (see Part 2), you will avoid getting this window incessantly popping up and keeping you stuck in a vicious error loop:
To disable the auto-debugging:
- Right click to ‘Inspect Element’, or click on F12 Developer Tools from the gear icon to open the Developer Tools pane
- Click on the "Debugger" tab
- Click on the Stop sign icon
- Select "Never break on exceptions"
Step 2
-
Once you are done with Part 1, make sure that debugging is enabled. To do this, you may go to:
-
Tools > Internet Options.
-
Select the Advanced tab.
-
Scroll down to the Browsing section and (if they aren’t already) uncheck the boxes labeled:
- Disable script debugging (Internet Explorer)
- Disable script debugging (other)
After you have taken these steps, you are finally ready to debug.
Step 3
If you want to figure out why something like a button is not working, you can:
-
Add an event breakpoint
-
Choose from the list of events (in this instance, click)
-
Proceed to test the click and step through the logic
If you want to step through your code more thoroughly and inspect a JS file line-by-line to find where the error occurs, you can also add a breakpoint to a specific line to stop execution. To do this, simply find the line of JS you want to target and click on it. It will now stop execution at this point.
At this point, it should hopefully be easy to trace the origin of the problem and see where the failure occurs. However, if that is not the case, it would be a good idea to look at the errors on the console again and double-check that it isn’t just an issue of a polyfill not applying (if you suspect it is, try adding the polyfill somewhere in the script to test), or if there is some outside script affecting the execution of a function.
The IE11 Best Practice: Regularly Monitor Cross-Browser Usage and Discuss It with Stakeholders
If you or your team haven’t established whether or not you are expected to support IE11, it is important to do so as soon as possible. If you are lucky, you may not have to, and this can free up your time to do more important work for your stakeholders. You want to maximize your time and deliver the best possible product, so spending a significant amount of time debugging in IE11 should be avoided if it’s not necessary.
On the other hand, if you are expected to support IE11, this would be an important time to set a best practice and get context for why IE11 needs to be supported. This would be a good opportunity to check in with your stakeholders on cross-browser usage and what percentage of the user base is on IE11. Since this can vary widely from less than one percent to 50 percent, it is important to know this and to keep an eye on it, especially since it may lessen as the years pass. For example, while supporting IE11 was important for your web application five years ago when the IE11 user base was 33 percent, it may no longer be the case if that user base has shrunk to 1 percent in the present day. At this point, it may be important for your stakeholders to re-evaluate the costs of maintaining IE11 compatibility vs. the value that user base brings to their goals.
If you and/or your stakeholder discover that your user base for IE11 is still statistically significant and can affect key factors such as revenue and other organizational goals, then it is vital that you provide IE11 users with either the same exact experience as users of other browsers, or an adequate alternative that allows them to accomplish their goals. According to W3Counter, the current market share for IE11 is 3 percent. This may not be significant, but if you were to make a rough analogy, Amazon.com in 2018 had an annual revenue of 241.5 billion dollars (Source). If 3 percent of their shoppers were Internet Explorer 11 users, that would be around 7.2 billion dollars of annual revenue lost if their services were not available to this specific subgroup of users.
Conclusion
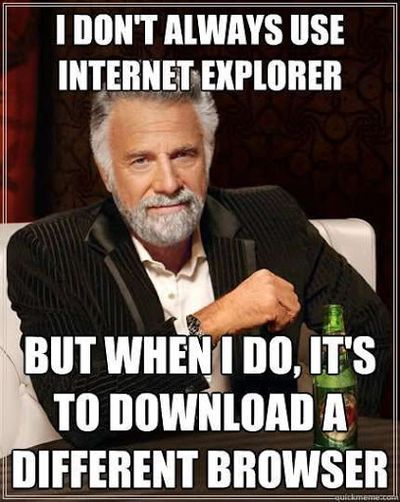
Popular memes and jokes aside, it's important to still retain the common pitfalls of developing for IE11 compatibility.
Source: Ranker
IE-themed jokes aside, this browser still covers a statistically significant percentage of the current market share (as of the publication of this post) and front-end engineers would do well to recognize its caveats in order to solve problems quickly and deliver a great product to stakeholders.